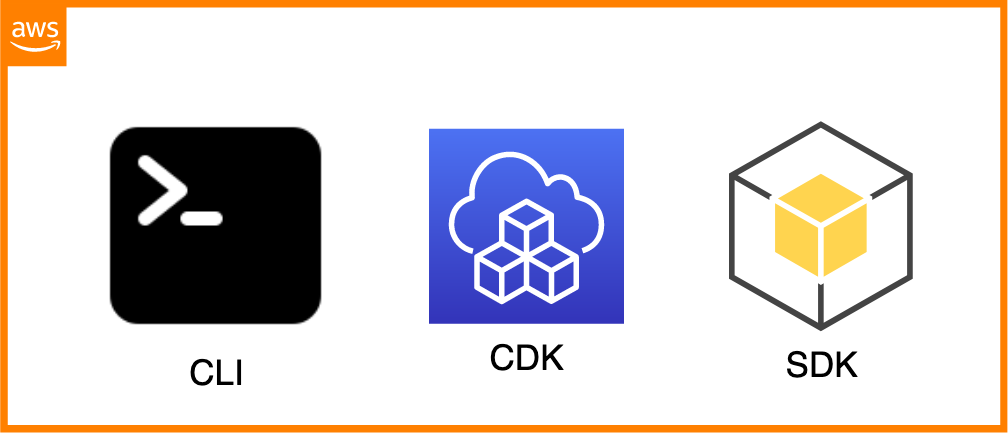
Overview
In this post, I will present differences in AWS IoT infrastructure management using CLI, CDK, and SDK.
As an example, we will create an IoT Thing using all of those approaches.
Setup
CLI
The AWS Command Line Interface (AWS CLI) enables interaction with AWS services using commands invoked in a shell.
Creating an IoT Thing using the AWS CLI is simple:
|
|
We can check the outcome by invoking the following command:
|
|
Pros of using CLI:
- a great way of learning about the AWS services by invoking low-level calls
- easy way to automate simple setup by executing CLI calls from a Bash script
Cons:
- we are responsible for maintaining the state of our assets (a sample IoT Thing was created and CLI “forgot” about it)
- deployment of a complex solution using CLI is hard to develop and maintain
CDK
The AWS Cloud Development Kit (AWS CDK) defines cloud infrastructure as code.
Before we can create our IoT Thing we need to make the CDK app.
Create the CDK app
|
|
Update the code
Open the ./cdk_iot_thing/cdk_iot_thing/cdk_iot_thing_stack.py
and replace it with the following sample:
|
|
Deploy
To actually create our IoT Thing we need to deploy the CDK App:
|
|
Pros of using CDK:
- CDK (actually the CloudFormation under the hood) maintains the state of our environment
- enables efficient management of complex systems
Cons:
- CDK requires an initial setup of the development environment
- steeper learning curve than for CLI (you need to learn the structure of AWS services and concepts related to the CDK itself)
- CDK does not support every action required to set up an Internet of Things environment
SDK
AWS SDK for Python (Boto3) allows the management of AWS services using Python script.
Some initial setup is required when working with the SDK:
|
|
Then we can actually create our IoT Thing:
|
|
Pros of using SDK:
- allows for the full control of the AWS environment (not limited to the CloudFormation functionalities like CDK)
- enables automated deployments and configuration of edge and cloud infrastructure
Cons:
- similarly to the CLI, we are responsible for maintaining the state of our assets (but that state management is easier than when using CLI)
- steeper learning curve than for CLI (you need to learn SDK and Python language)
Cleanup
Now let’s remove our IoT Things and observe differences in the state management between the CLI, CDK, and SDK.
CLI
To remove an IoT Thing using the CLI we need to know its name. In huge-scale automated deployments, keeping track of managed IoT Things is not a trivial task and CLI does not support us much.
|
|
CDK
To remove the IoT Thing managed by the CDK App, we need to destroy that App.
The name of IoT Thing is known by the CDK App, so we do not have to remember or store it anywhere - state management is a huge benefit of using CDK.
In terminal execute:
|
|
SDK
When we use the SDK, we can list all IoT Things and destroy them in the loop.
That might be a bit risky operation, but it is very efficient (provided that we know what we are doing).
SDK does not manage the state of our environment, but we can leverage Python to build a custom management logic.
|
|
Conclusion
I hope that this simplified example presented the differences when working with CLI, CDK, and SDK. Every tool requires a bit different approach.
Personally, I prefer to use CDK to manage complex IoT deployments at AWS. To fine-tune setup and invoke specific functions, I support CDK with SDK.
