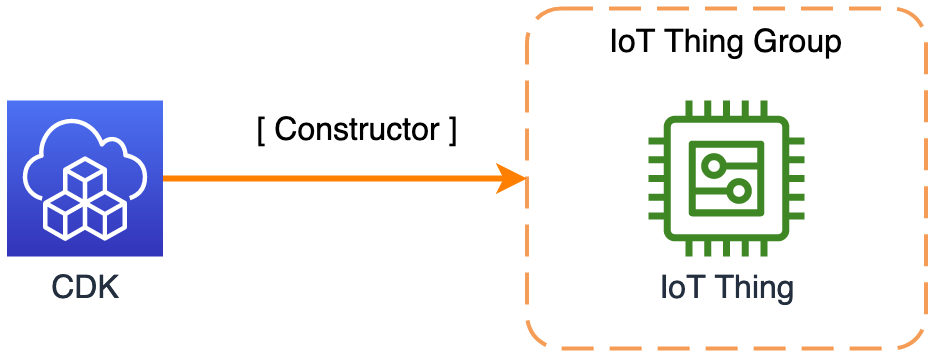
How to create an IoT Thing Group using CDK?
By default, we can not manage IoT Thing Groups using AWS Cloud Development Kit (CDK). CDK deploys infrastructure using CloudFormation and CloudFormation does not support Thing Groups.
Today I want to share an elegant solution for this challenge.
CDK Constructor
I propose to create a custom CDK Constructor. Constructor represents a specific resource and enables the management of the corresponding AWS infrastructure. This way we can encapsulate complex logic with easy to use abstraction.
Please check the AWS documentation for additional information.
How Constructor manages infrastructure not supported by the CloudFormation?
Under the hood, CDK creates an AWS Lambda-backed custom resource that calls AWS APIs directly.
Custom Resources are often used in the CloudFormation templates. In my opinion, CDK encapsulates them in a very elegant way.
Let’s start by defining how we would like to manage the IoT Thing Group using CDK App.
I propose the following approach:
|
|
That looks good, but how can we implement this Constructor?
Implementation
This is a sample implementation of a CDK Constructor that enables the management of IoT Thing Groups.
We implemented different AWS API calls for on_update
and on_delete
actions.
Additionally, we exposed the ARN (Amazon Resource Name) of the managed resource, so we can use it in our CDK App.
|
|
CDK Deployment
To create our IoT Thing Group, we need to deploy this CDK App:
|
|
During deployment, we can review changes to be introduced in our infrastructure.
As you can see, CDK will create an IAM Policy that allows for the iot:CreateThingGroup
and iot:DeleteThingGroup
actions.
That IAM Policy will be used by a Lambda function to manage our resources (the IoT Thing Group in this case).
Deployment review
After a few seconds, our stack will be created. We can confirm that the Name
and `ARN`` of our IoT Thing Group are accessible from the CDK App.
That is very convenient especially when one team implements Constructors and some other team uses those Constructors to deploy AWS infrastructure.
Let’s check our IoT Thing Group in the AWS Console:
Once we are in the AWS Console, we can check the CloudFormation stack created by our CDK App:
There is no IoT Thing Group resource, only the Custom Resource that represents the CDK Constructor we created.
Summary
This way we created an IoT Thing Group (a resource that is not supported by CDK/CloudFormation) using our CDK App.
It is just a simple example that shows how can we encapsulate complex logic and low-level AWS API calls using easy to use high-level object - a CDK Constructor.
